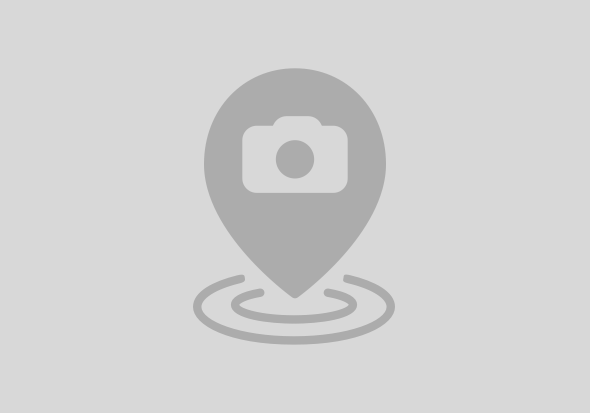
@JSInject(targetScreen = "sales")
public InputStream[] injectJS() {
return new InputStream[] {this.getClass().getResourceAsStream("/resources/salesInject.js")};
}
// Add the input field
$(document).ready(function () {
$('.customerInfoContainer').after($('<div style="position: relative; right: 0; top: 0; background-color: #FFF; padding: 5px; border: 1px solid #000; width: 25%; height: 68%; font-size: small; margin-left: 5px; margin-right: 5px; margin-top: 5px; float:left" id="customerAgeDiv">Age of Customer: <input type="number" id="customerAge"></div>'));
});
// monkey path the original execute method.
var origMethod = BasicReceiptActionCommand.prototype.execute;
// add our own code and call the original method we monkey patched.
BasicReceiptActionCommand.prototype.execute = function (a, b, c) {
if (a.entity === "createReceipt") {
var receiptKey = $('#receiptEntityKey').val();
var requestBody = '{' + "\"customerAge\" : " + $("#customerAge").val() + ' }';
$.ajax({
type: "POST",
url: "PluginServlet?action=customerAge&receiptId="+encodeURIComponent(receiptKey),
data: requestBody,
contentType: "application/json",
dataType: "json",
success: function(result) {
if (result && result["status"] == 1) {
$("#customerAge").val(0);
}
}
});
}
origMethod.call(this, a, b, c);
};
if (a.entity === "createReceipt") {
var receiptKey = $('#receiptEntityKey').val();
var requestBody = '{' + "\"customerAge\" : " + $("#customerAge").val() + ' }';
$.ajax({
type: "POST",
url: "PluginServlet?action=customerAge&receiptId="+encodeURIComponent(receiptKey),
data: requestBody,
contentType: "application/json",
dataType: "json",
success: function(result) {
if (result && result["status"] == 1) {
$("#customerAge").val(0);
}
}
});
origMethod.call(this, a, b, c);
@ListenToExit(exitName="PluginServlet.callback.post")
public void pluginServletPost(Object caller, Object[] args) {
HttpServletRequest request = (HttpServletRequest)args[0];
HttpServletResponse response = (HttpServletResponse)args[1];
RequestDelegate.getInstance().handleRequest(request, response);
}
/**
* @author Robert Zieschang
*/
public class RequestDelegate {
private static final Logger logger = Logger.getLogger(RequestDelegate.class);
private CustomerAgeDao customerAgeDao;
private static RequestDelegate instance = new RequestDelegate();
public static synchronized RequestDelegate getInstance() {
if (instance == null) {
instance = new RequestDelegate();
}
return instance;
}
private RequestDelegate() {
this.customerAgeDao = new CustomerAgeDao();
}
public void handleRequest(HttpServletRequest request, HttpServletResponse response) {
String urlMethod = request.getMethod();
String action = request.getParameter("action");
JSONObject responseObj = new JSONObject();
if ("POST".equals(urlMethod)) {
JSONObject requestBody = this.getRequestBody(request);
switch(action) {
case "customerAge":
String receiptKey = request.getParameter("receiptId");
customerAgeDao.save(new CustomerAgeDto(receiptKey, requestBody.getInt("customerAge")));
responseObj.put("status", 1);
break;
}
} else if ("GET".equals(urlMethod)) {
}
if(!responseObj.isEmpty()) {
try (OutputStreamWriter osw = new OutputStreamWriter(response.getOutputStream())) {
osw.write(responseObj.toString());
} catch (IOException e) {
logger.severe("Error while Processing Request");
}
}
}
private JSONObject getRequestBody(HttpServletRequest request) {
StringBuilder sb = new StringBuilder();
try {
BufferedReader br = request.getReader();
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line);
}
} catch (IOException e) {
logger.severe("Error while parsing JSON Request body");
}
return JSONObject.fromObject(sb.toString());
}
}
if ("POST".equals(urlMethod)) {
JSONObject requestBody = this.getRequestBody(request);
switch(action) {
case "customerAge":
String receiptKey = request.getParameter("receiptId");
customerAgeDao.save(new CustomerAgeDto(receiptKey, requestBody.getInt("customerAge")));
responseObj.put("status", 1);
break;
}
} else if ("GET".equals(urlMethod)) {
}
success: function(result) {
if (result && result["status"] == 1) {
$("#customerAge").val(0);
}
}
public class CustomerAgeDao {
private static final Logger logger = Logger.getLogger(CustomerAgeDao.class);
private static final String TABLE_NAME = "HOK_CUSTOMERAGE";
private static final String QUERY_CREATE_TABLE = "CREATE TABLE " + TABLE_NAME + " ("
+ "RECEIPTKEY varchar(100) not null PRIMARY KEY,"
+ "CUSTOMERAGE INT not null)";
private static final String QUERY_INSERT_ROW = "INSERT INTO " + TABLE_NAME + " VALUES(?,?)";
private static final String QUERY_UPDATE_ROW = "UPDATE " + TABLE_NAME
+ " SET RECEIPTKEY = ?2 WHERE CUSTOMERAGE = ?1";
// query for find all
private static final String QUERY_FIND_ALL = "SELECT RECEIPTKEY, CUSTOMERAGE FROM " + TABLE_NAME;
// query for find one
private static final String QUERY_FIND_ONE = "SELECT RECEIPTKEY, CUSTOMERAGE FROM " + TABLE_NAME
+ " where RECEIPTKEY = ?";
// query to drop one
private static final String QUERY_DROP_ONE = "DELETE FROM " + TABLE_NAME + " WHERE RECEIPTKEY = ?";
public void setupTable() {
CDBSession session = CDBSessionFactory.instance.createSession();
try {
session.beginTransaction();
EntityManager em = session.getEM();
Query q = em.createNativeQuery(QUERY_CREATE_TABLE);
q.executeUpdate();
session.commitTransaction();
logger.info("Created table " + TABLE_NAME);
} catch (Exception e) {
session.rollbackDBSession();
logger.info("Error or table " + TABLE_NAME + " already existing");
} finally {
session.closeDBSession();
}
}
private void save(CustomerAgeDto customerAge, boolean isAlreadyInDB) {
CDBSession session = CDBSessionFactory.instance.createSession();
String query = isAlreadyInDB ? QUERY_UPDATE_ROW : QUERY_INSERT_ROW;
try {
session.beginTransaction();
EntityManager em = session.getEM();
Query q = em.createNativeQuery(query);
q.setParameter(1, customerAge.getReceiptKey());
q.setParameter(2, customerAge.getCustomerAge());
q.executeUpdate();
session.commitTransaction();
} catch (Exception e) {
session.rollbackDBSession();
logger.info("Could not create CustomerAge");
logger.info(e.getLocalizedMessage());
} finally {
session.closeDBSession();
}
}
public List<CustomerAgeDto> findAll() {
CDBSession session = CDBSessionFactory.instance.createSession();
ArrayList<CustomerAgeDto> resultList = new ArrayList<>();
try {
session.beginTransaction();
EntityManager em = session.getEM();
Query q = em.createNativeQuery(QUERY_FIND_ALL);
@SuppressWarnings("unchecked")
List<Object[]> results = q.getResultList();
if (results.isEmpty()) {
// return an empty list rather than null
return resultList;
}
for (Object[] resultRow : results) {
resultList.add(new CustomerAgeDto((String) resultRow[0], (Integer) resultRow[1]));
}
} catch (Exception e) {
logger.info("Error while getting results from table " + TABLE_NAME);
} finally {
session.closeDBSession();
}
return resultList;
}
public CustomerAgeDto findOne(String inReceiptKey) {
CDBSession session = CDBSessionFactory.instance.createSession();
CustomerAgeDto customerAge = new CustomerAgeDto();
try {
EntityManager em = session.getEM();
Query q = em.createNativeQuery(QUERY_FIND_ONE);
q.setParameter(1, inReceiptKey);
@SuppressWarnings("unchecked")
List<Object[]> results = q.getResultList();
if (results.isEmpty()) {
// return empty dto
return customerAge;
}
customerAge.setReceiptKey((String) results.get(0)[0]);
customerAge.setCustomerAge((Integer) results.get(0)[1]);
} catch (Exception e) {
logger.info("Error while getting " + inReceiptKey + " from table " + TABLE_NAME);
} finally {
session.closeDBSession();
}
return customerAge;
}
public void dropOne(String inReceiptKey) {
CDBSession session = CDBSessionFactory.instance.createSession();
try {
session.beginTransaction();
EntityManager em = session.getEM();
Query q = em.createNativeQuery(QUERY_DROP_ONE);
q.setParameter(1, inReceiptKey);
q.executeUpdate();
session.commitTransaction();
} catch (Exception ex) {
} finally {
session.closeDBSession();
}
}
public void dropAll() {
List<CustomerAgeDto> list = this.findAll();
for (CustomerAgeDto entry : list) {
this.dropOne(entry.getReceiptKey());
}
}
public void save(CustomerAgeDto customerAge) {
CustomerAgeDto customerAgeInDb = this.findOne(customerAge.getReceiptKey());
boolean isAlreadyInDb = customerAge.getReceiptKey().equals(customerAgeInDb.getReceiptKey());
// check if entity is already in database, so that we update rather than insert.
this.save(customerAge, isAlreadyInDb);
}
public class CustomerAgeDto {
private String receiptKey;
private Integer customerAge;
public CustomerAgeDto() {
}
public CustomerAgeDto(String inReceipKey, Integer inCustomerAge) {
this.receiptKey = inReceipKey;
this.customerAge = inCustomerAge;
}
public String getReceiptKey() {
return receiptKey;
}
public void setReceiptKey(String receiptKey) {
this.receiptKey = receiptKey;
}
public Integer getCustomerAge() {
return customerAge;
}
public void setCustomerAge(Integer customerAge) {
this.customerAge = customerAge;
}
}
@ListenToExit(exitName="BusinessOneServiceWrapper.beforePostInvoiceRequest")
public void enlargeB1iMessage(Object caller, Object[] args) {
ReceiptDTO receiptDto = (ReceiptDTO) args[0];
PostInvoiceType request = (PostInvoiceType) args[1];
CustomerAgeDto customerAge = this.customerAgeDao.findOne(receiptDto.getKey());
if (null != customerAge.getReceiptKey()) {
GenericValues.KeyValPair keyValPair = new GenericValues.KeyValPair();
keyValPair.setKey("customerAge");
keyValPair.setValue(customerAge.getCustomerAge().toString());
if (null == request.getGenericValues()) {
request.getSale().getDocuments().setGenericValues(new GenericValues());
}
request.getSale().getDocuments().getGenericValues().getKeyValPair().add(keyValPair);
args[1] = request;
}
}
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.