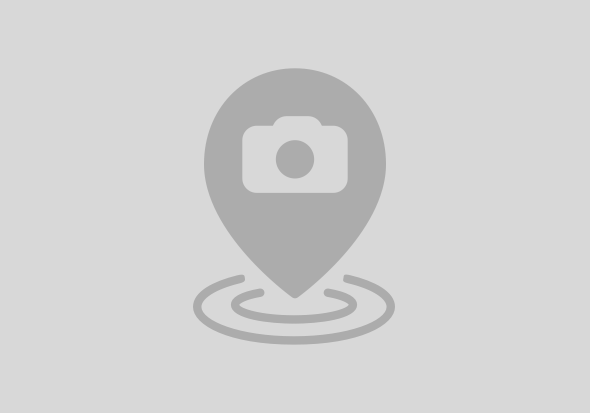
In a previous blog I http://scn.sap.com/community/crm/blog/2013/02/07/crmorderread-simple-example-for-those-new-to-crm-an... gave a very simple example of how to read an order using CRM_ORDER_READ.
Then in another blog I explained how to UPDATE an order: http://scn.sap.com/community/crm/blog/2013/02/07/crmordermaintain-simple-example-to-update-an-order-...
Now I’ll give a simple example of how to create an order using CRM_ORDER_MAINTAIN.
This blog will show you how to CREATE an order from scratch using ABAP.
I’ve tried to make this as simple as possible but while including the most important (useful) information.
For this example I will create an order with two tables populated: orderadm_h and opport_h (which signifies that this is an OPPORTUNITY that I’m creating)
include crm_object_names_con.
data:
* All the tables to be created
lt_orderadm_h type crmt_orderadm_h_comt,
ls_orderadm_h type crmt_orderadm_h_com,
lt_opport_h type crmt_opport_h_comt,
ls_opport_h type line of crmt_opport_h_comt,
* Other important things
lt_input_fields type crmt_input_field_tab,
ls_input_fields type crmt_input_field,
lt_nametab type crmt_input_field_names_tab,
ls_nametab type crmt_input_field_names,
lt_save_guid type crmt_object_guid_tab,
ls_save_guid type crmt_object_guid,
lt_saved_objects type crmt_return_objects,
ls_saved_objects type crmt_return_objects_struc,
lv_lin type i,
lv_order_object_id type crmt_object_id,
lv_order_object_guid type crmt_object_guid32.
* Calculate the “handle” that will join all these attributes together
call function 'CRM_INTLAY_GET_HANDLE'
importing
ev_handle = ls_input_fields-ref_handle.
* 1. Populate orderadm_h with the process type and description
ls_orderadm_h-handle = ls_input_fields-ref_handle.
ls_orderadm_h-process_type = ‘process type string’.
ls_orderadm_h-description = ‘this is a description’.
ls_orderadm_h-mode = 'A'.
append ls_orderadm_h to lt_orderadm_h.
* 2. Prepare table first.
* The lines you enter into table lt_nametab MUST be entered (appended)
* in alphabetical order, as lt_nametab is sorted.
* See here that the 3 entried are the three attributes you populated in ls_orderadm_h above.
ls_nametab = 'DESCRIPTION'.
append ls_nametab to lt_nametab.
ls_nametab = 'MODE'.
append ls_nametab to lt_nametab.
ls_nametab = 'PROCESS_TYPE'.
append ls_nametab to lt_nametab.
* 3. Populate lt_input_fields now
* You need to add this table that you want to create to the input fields.
ls_input_fields-ref_kind = 'A'.
ls_input_fields-objectname = 'ORDERADM_H'.
ls_input_fields-field_names[] = lt_nametab[].
insert ls_input_fields into table lt_input_fields.
* 4. Prepare the next table you want to create for this opportunity: opport_h
clear: ls_nametab, lt_nametab[].
* 5. Populate ls_opport_h with appropriate values (see examples or attributes to populate)
ls_opport_h-ref_handle = ls_input_fields-ref_handle.
ls_opport_h-exp_revenue = iv_expt_sales.
ls_opport_h-startdate = iv_sc_sdate.
ls_opport_h-expect_end = iv_sc_cdate.
ls_opport_h-curr_phase = iv_sales_stage.
ls_opport_h-probability = iv_chanceofsuc.
ls_opport_h-source = iv_origin.
ls_opport_h-type = iv_serv_line.
ls_opport_h-mode = 'A'.
append ls_opport_h to lt_opport_h.
* 6. Prepare the lt_nametab table – remember to be alphabetical again!
ls_nametab = 'CURR_PHASE'.
append ls_nametab to lt_nametab.
ls_nametab = 'EXPECT_END'.
append ls_nametab to lt_nametab.
ls_nametab = 'EXP_REVENUE'.
append ls_nametab to lt_nametab.
ls_nametab = 'MODE'.
append ls_nametab to lt_nametab.
ls_nametab = 'PROBABILITY'.
append ls_nametab to lt_nametab.
ls_nametab = 'SOURCE'.
append ls_nametab to lt_nametab.
ls_nametab = 'STARTDATE'.
append ls_nametab to lt_nametab.
ls_nametab = 'TYPE'.
append ls_nametab to lt_nametab.
* 7. Add to lt_input fields the new table you want to create.
ls_input_fields-ref_kind = 'A'.
ls_input_fields-objectname = 'OPPORT_H'.
ls_input_fields-field_names[] = lt_nametab[].
insert ls_input_fields into table lt_input_fields.
* 8. You’re ready to call crm_order_maintain now
call function 'CRM_ORDER_MAINTAIN'
exporting
it_opport_h = lt_opport_h
changing
ct_orderadm_h = lt_orderadm_h
ct_input_fields = lt_input_fields
exceptions
error_occurred = 1
document_locked = 2
no_change_allowed = 3
no_authority = 4
others = 5.
* 9. Read the table that has been changed and get the GUID that was created.
case sy-subrc.
when '0'.
read table lt_orderadm_h into ls_orderadm_h index 1.
ls_save_guid = ls_orderadm_h-guid.
append ls_save_guid to lt_save_guid.
endcase.
* 10. SAVE the changes (all creations must be saved and committed before they exist in CRM)
call function 'CRM_ORDER_SAVE'
exporting
it_objects_to_save = lt_save_guid
importing
et_saved_objects = lt_saved_objects
exceptions
document_not_saved = 1
others = 2.
case sy-subrc.
when '0'.
clear lv_lin.
describe table lt_saved_objects lines lv_lin.
if lv_lin = 1.
read table lt_saved_objects into ls_saved_objects index 1.
lv_order_object_guid = ls_saved_objects-guid.
lv_order_object_id = ls_saved_objects-object_id.
* 11. Call the function to COMMIT the changes to CRM.
call function 'BAPI_TRANSACTION_COMMIT'.
endif.
endcase.
*Your new opportunity will now exist in CRM – use CRM_ORDER_READ to read it, using its GUID
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
6 | |
4 | |
1 | |
1 | |
1 |