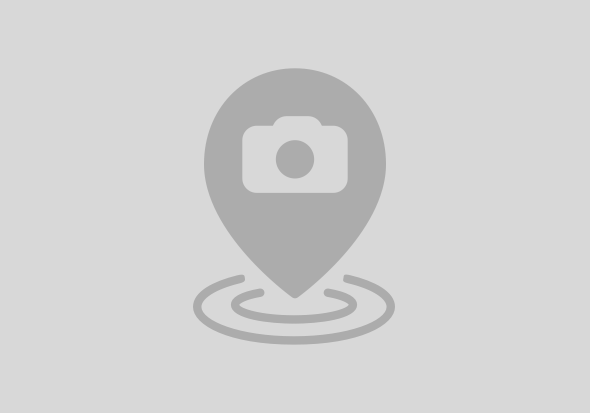
{
"Caption": "VideoPlayer",
"Controls": [
{
"Sections": [
{
"Class": "VideoPlayer",
"Control": "VideoPlayer",
"ExtensionProperties": {
"videoURL": "http://commondatastorage.googleapis.com/gtv-videos-bucket/sample/BigBuckBunny.mp4"
},
"EmptySection": {
"FooterVisible": false
},
"Height": 1000,
"Module": "extension-VideoPlayer",
"Visible": true,
"_Name": "SectionExtension0",
"_Type": "Section.Type.Extension"
}
],
"_Name": "SectionedTable0",
"_Type": "Control.Type.SectionedTable"
}
],
"_Name": "VideoPlayer",
"_Type": "Page"
}
extension-VideoPlayer
- contols
- VideoPlayer.ts
import { IControl } from 'mdk-core/controls/IControl';
import { BaseObservable } from 'mdk-core/observables/BaseObservable';
import { IContext } from 'mdk-core/context/IContext';
import * as app from 'tns-core-modules/application';
import { screen } from 'tns-core-modules/platform';
export class VideoPlayer extends IControl {
private _observable: BaseObservable;
private _videoURL: string;
private _avplayerViewController: AVPlayerViewController;
private _avPlayer: AVPlayer;
public initialize(props) {
super.initialize(props);
this._avplayerViewController = AVPlayerViewController.new();
this._avplayerViewController.view.frame = CGRectMake(0, 0, 0, 2000);
this._avplayerViewController.entersFullScreenWhenPlaybackBegins = true;
this._avplayerViewController.showsPlaybackControls = true;
const sVideoURL = this.definition().data.ExtensionProperties.videoURL;
this.valueResolver().resolveValue(sVideoURL).then((resolvedValue) => {
this._videoURL = resolvedValue;
this._avPlayer = AVPlayer.playerWithURL(NSURL.URLWithString(this._videoURL));
this._avplayerViewController.player = this._avPlayer;
if (this._avPlayer){
this._avPlayer.play();
}
});
}
public view() {
return this._avplayerViewController.view;
}
public viewIsNative() {
return true;
}
public observable() {
if (!this._observable) {
this._observable = new BaseObservable(this, this.definition(), this.page());
}
return this._observable;
}
public setContainer(container: IControl) {
// do nothing
}
public setValue(value: any, notify: boolean, isTextValue?: boolean): Promise<any> {
return Promise.resolve();
}
public onPageLoaded() {
app.on(app.orientationChangedEvent, this._fireOrientationChangedEvent, this);
}
public onPageUnloaded(pageExists) {
app.off(app.orientationChangedEvent);
}
private _fireOrientationChangedEvent(data: any) {
this.resetSize();
}
private resetSize() {
if (app.ios) {
const width = screen.mainScreen.heightDIPs > screen.mainScreen.widthDIPs ? screen.mainScreen.widthDIPs : screen.mainScreen.heightDIPs;
const height = screen.mainScreen.heightDIPs > screen.mainScreen.widthDIPs ? screen.mainScreen.heightDIPs : screen.mainScreen.widthDIPs;
if (this._avplayerViewController) {
this._avplayerViewController.view.frame = CGRectMake(0, 0, width, height);
}
}
}
}
Fix for App Transport Issue
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
17 | |
15 | |
11 | |
11 | |
9 | |
8 | |
8 | |
7 | |
7 | |
7 |