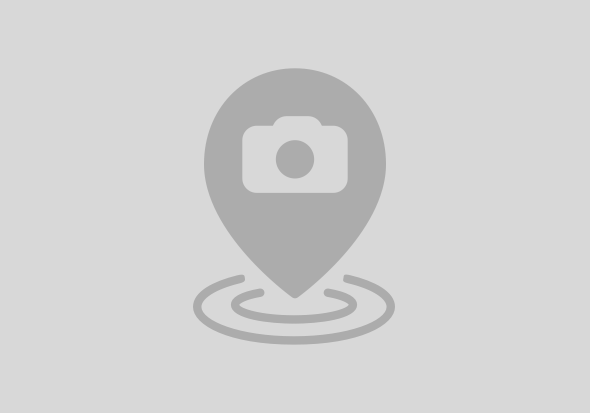
import sys
sys.path.append(r"C:\Program Files\SAP Predictive Analytics\Desktop\Automated\EXE\Clients\Python35")
import os
os.environ['PATH'] = r"C:\Program Files\SAP Predictive Analytics\Desktop\Automated\EXE\Clients\CPP"
AA_DIRECTORY = "C:\Program Files\SAP Predictive Analytics\Desktop\Automated"
import aalib
class DefaultContext(aalib.IKxenContext):
def __init__(self):
super().__init__()
def userMessage(self, iSource, iMessage, iLevel):
print(iMessage)
return True
def userConfirm(self, iSource, iPrompt):
pass
def userAskOne(iSource, iPrompt, iHidden):
pass
def stopCallBack(iSource):
pass
frontend = aalib.KxFrontEnd([])
factory = frontend.getFactory()
context = DefaultContext()
factory.setConfiguration("DefaultMessages", "true")
config_store = factory.createStore("Kxen.FileStore")
config_store.setContext(context, 'en', 10, False)
config_store.openStore(AA_DIRECTORY + "\EXE\Clients\CPP", "", "")
config_store.loadAdditionnalConfig("KxShell.cfg")
# LOAD Model
folder_name = r"O:\MODULES_PA/PYTHON_API/MY_MODELS"
model_name = "My Classification Model"
store = factory.createStore("Kxen.FileStore")
store.openStore(folder_name, "", "")
model = store.restoreLastModelD(model_name)
help(model.generateCode)
output_file = "MY_CLASSIF_EQUATION.java"
output_folder = r"O:\MODULES_PA/PYTHON_API/MY_MODELS"
model.generateCode("JAVA", output_folder, output_file)
help(model.generateCode2)
# Set the Key and Target Columns
target_col = "IS_FRAUD"
key_col = "CLAIM_ID"
# Set the Roles
model.getParameter("")
variables = model.getParameter("Protocols/Default/Variables")
variables.setAllValues("Role", "input")
variables.setSubValue(target_col + "/Role", "target")
variables.setSubValue("KxIndex/Role", "skip")
variables.setSubValue("KxIndex/KeyLevel", "0")
variables.setSubValue(key_col + "/Role", "skip")
variables.setSubValue(key_col + "/KeyLevel", "1")
model.validateParameter()
output_folder = r"O:\MODULES_PA/PYTHON_API/MY_MODELS"
output_file = "DETECT_FRAUD_EQUATION.sql"
table_name = "NEW_CLAIMS"
key_col = "CLAIM_ID"
model.generateCode2("HANA", output_folder, output_file, "", table_name, key_col)
t = model.getTransformInProtocol("Default", 0)
t.getParameter("")
t.changeParameter("Parameters/ExtraMode", "Advanced Apply Settings")
t.validateParameter()
# Get Long Names For Output Columns
model.getParameter("")
model.changeParameter("Parameters/CodeGeneration/UseVarNameAsAlias", "true")
model.validateParameter()
# Prepare Settings Parameter
target_col = "is_fraud"
d_path = "Protocols/Default/Transforms/Kxen.RobustRegression/Parameters/ApplySettings/Supervised/%s" % target_col
settings = model.getParameter(d_path)
# Decision
flag = settings.getSubParameter("PredictedRankCategories")
flag.removeAll()
flag.insert("1")
# Probability Decision
flag = settings.getSubParameter("PredictedRankProbabilities")
flag.removeAll()
flag.insert("1")
model.validateParameter()
# Individual Contributions
t.getParameter("")
d_path = "Parameters/ApplySettings/Supervised/%s/Contribution" % target_col
t.changeParameter(d_path, "all")
t.validateParameter()
output_file = "DETECT_FRAUD_EQUATION_ADV.sql"
model.generateCode2("HANA", output_folder, output_file, "", table_name, key_col)
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
21 | |
13 | |
11 | |
10 | |
9 | |
8 | |
7 | |
7 | |
7 | |
7 |