This blog post details my
100-day low-code, no-code journey, and hopefully provides some tips and tricks for you.
In the
last post, I described setting up my mobile device to view my apps, understanding the interface, and experimenting with simple data that was stored on the device.
In the current post, I'll describe working with data, what is happening behind the scenes, and how you can control what is happening in your app (with about as much code as an Excel formula).
Day 5 - Connecting to API |
I wanted to use this free MLB Data API to retrieve team, player and stats information.
Data Resource
Though it was one API set, I would create a data resource for each API endpoint I needed. I started with the list of teams.
I went to the DATA tab at the top, and created a new REST

On the base screen I gave the data resource a name/description, and gave the base URL. I have options for the following:
- HTTP Headers: For example, if I want to return JSON format and need to send an ACCEPT header.
- URL Placeholder: If I want part of the URL to be dynamic, for example, if the URL includes a team name to return its players, I can provide that later when I actually make the call.
- Query Parameters
Since I want a collection of teams, I set up the call to return the list of teams by providing the path for the API call. I could have defined the query parameters with the query parameters function instead of hard-coding in the path – and I probably should, because later I may need to make calls for other leagues, years, and sort orders and want to set the query parameters dynamically.
Since the API did not return a list, but embedded the list inside the response, I had to provide the path to the list within the response.
team_all_season.queryResults.row
So the collection configuration looked like this:

I then went to the Test tab and tested the API.

After getting the data successfully, I click
SET SCHEMA FROM RESPONSE to set the schema, which AppGyver will later use in helping me bind the fields to the UI.
Data Variable
In order to use the data, we need to store it in a data variable with the schema we just created. Data variables are only usable within the page for which they are created.
On the page, I went to the
Variables section, and clicked
Add Data Variable, and added a variable based on the data resource for the teams API.

I set is to be for a collection of data records, and that was it.

UI
Now I wanted to see the data, so I went back to the view of the page, and added a dropdown field, since I wanted the user to be able to select a team. I set the following:

That's all I needed to do, and I got a dropdown list.

Day 6 - Understand how API calls work |
So far we have not dealt with the Logic panel, and responding to events, and so far AppGyver handled all the event handling. But now we will look at what is happening.
I opened
Variables tab → Data Variables → Show Logic (bottom).
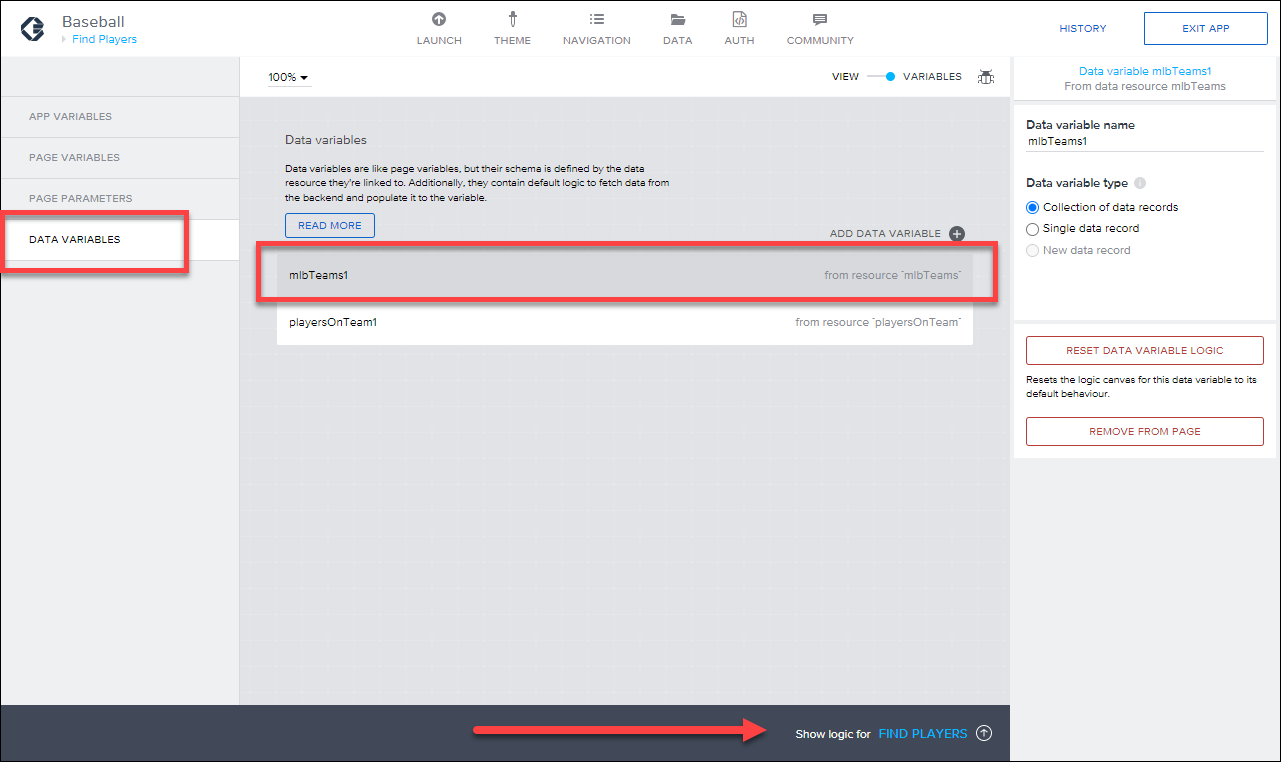
Now we can see what is going on.

- The page mounted (loaded) event is captured.
- The teams data resource is executed, and the data is retrieved.
- The retrieved data is placed in my data variable. Every bound to that variable will now display the data.
After the attempt to retrieve the data, the system waits 5 seconds (Delay) and then another attempt is made to retrieve the records, keeping the data up to date.
All of this helps to understand the flow, and lets us tweak it, which is what I did the next day.
Add list of players
I improved my app so that when someone selects a team, all the players are shown.
- I created another data resource called playersOnTeam, but this time I cleaned it up and defined the query parameters separately (though still static values).

- I created a data variable based on the data source above. I set it to a collection of records and set the team_id query parameter (which I said was mandatory but dynamic) to the page variable I created for storing the team ID from the dropdown field.

- I added a text field, and set it to repeat based on my data variable, and set the primary label to the name of the player.

And now I had a list of teams, and then when I chose one I could see all the players.

Day 7 - Tweaking my API call |
But the application wasn't exactly the way I wanted it, so I decided to try to tweak a few things, which is when I think you learn an application the best.
Get teams data once
By default, the API to retrieve the list of teams gets called every 5 seconds, to check for updates. But the list of major league baseball teams rarely changes (though it did change at the start of the season as the Cleveland team changed its name to the Guardians).
So I removed the loop to recall the API (defined with the data variable), and the list of teams is fetched only when the page is mounted. I might be able to do this only once when the application loads, but I'll leave this for another day.

Get players right away
When I select a team, it takes a few seconds for the list of players to update. Thats because by default the data gets updated only every 5 seconds, not when the selected team changes.
So I went to the logic panel for the dropdown field, and had the API for players called whenever the selected team page variable changed.

Everything is pretty intuitive, except when configuring the Set data variable flow function, you have to specify from where to get the data for the variable. This must be set to
Output value of another node → Get record collection (the previous node in the chain).
Put Players list in scroll region
I wanted to keep the dropdown list at the top, but let the user scroll through the list of players. I also wanted to automatically scroll back to the top when a user picked a new team.
I added a scroll view, but quickly learned that you had to turn off scrolling on the page and set a different setting for the scroll view to work. After this it worked nicely ... for the most part.

But now I want to have a button on the bottom of screen after the scroll view ... this I was confusing to me. But in the end, I simply added all the footer stuff at the end but inside the scroll view. So when the user finishes scrolling the list of players he also gets additional stuff.
I've seen people often want a footer, and I think this is possible, but I think it takes too much space, so I did not try, but I will in the future.
Wait for dropdown to close
In my mobile app, when I select a team, the team gets selected even before I close the select dial. I want it not to change until I close the dropdown selector, but I have not found a way to do this.
Let me know if you know ... in the meantime, I have opened up a
forum post.
Day 8 - API with special characters |
When I set up my API call to retrieve my list of players, everything worked fine in the web preview but the players did not display in the mobile app.
After a long debugging process, I realized that my API returned non-standard characters, and I assumed this was causing problems for me.

Luckily, the API had parameters for including/excluding specific fields from the response, so that is what I did with the 2 fields that had special characters. And then everything worked OK.
Some tips
The data resources have some cool features:
- You can have placeholders within the URL or for query parameters that can be dynamically assigned a value at the time of the API call.
- You can specify a path within the response where your data will be.
- When we have BTP destinations, it is expected that you will be able to do some post-processing on the response before sending to the data variable.
- You can specify default or initial values for the query parameters, but then let them be dynamically set.
But there's more ...
How data access works
There may be other ways to access data (e.g., manual HTTP request) but in AppGyver this is the basic way API calls are done:
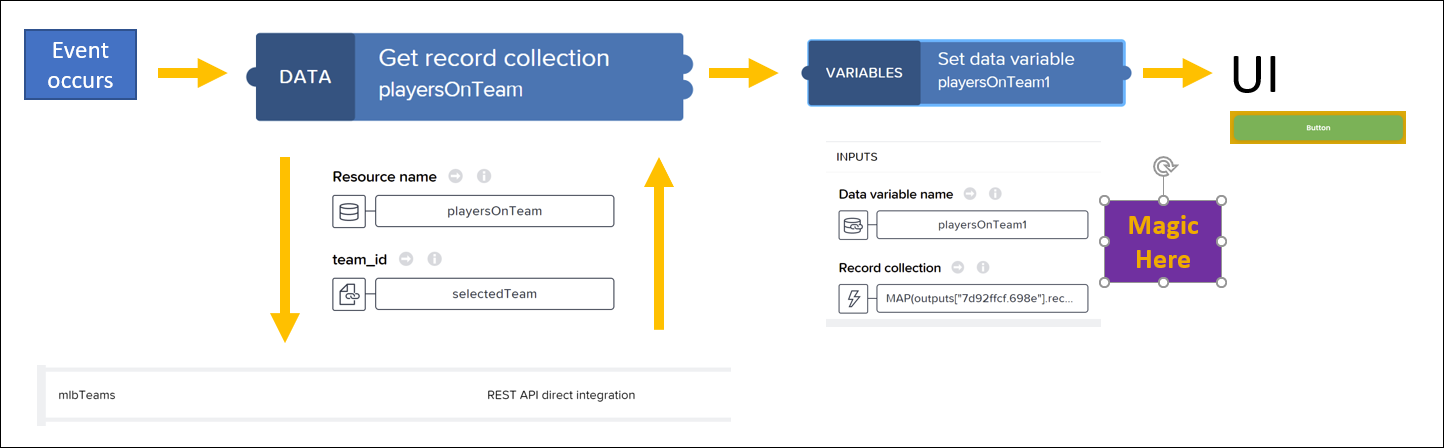
- An event occurs and is captured.
- A "Get record collection" flow function is run, which runs a specific data resource. Any required parameters are provided.
- The API call defined by the data resource is run, and the list of records is returned to the app.
- A "Set data variable" is loaded with the data. But here's where the magic happens, and you can control how the data is handled. More on this below.
- Any UI control bound to the data variable will be updated with the data.
Now, why is there
magic?
In the "Set data variable" function, generally you take the records as you received them, so you specify as the data binding
Output value of another node → Get record collection flow function → Records. (Most times this binding is created for you.)

But instead, we can intercept these records and do anything we want, creating totally new records or modifying the existing. So we could create a formula to create completely new records, remove some of the fields, filter the data, or change the values.
For example, I could say I want only names starting with C, and then rewrite the name to include the players jersey number.
MAP(SELECT(outputs["Get record collection"].records, SUBSTRING(item.name_first_last,0,1) == 'C'),DECODE_JSON('{"name_last_first" : "' + item.name_last_first + ' (' + item.jersey_number + ')"}'))
This is done by setting the data records with a formula. But you know, basically everything is a formula, it seems. When we were taking the records straight, we were essentially using a formula.
outputs["Get record collection"].records
Anyway, I think all this is cool, because I can more easily control what happens inside my AppGyver app
You can go all-in on all the low-code, no-code tools and take the 18-hour, 8-unit
low-code, no-code learning journey (SAP AppGyver, SAP Process Automation, SAP Work Zone) – and it includes a certification exam, if you are interested (fee) – which is part of the new
SAP Learning.