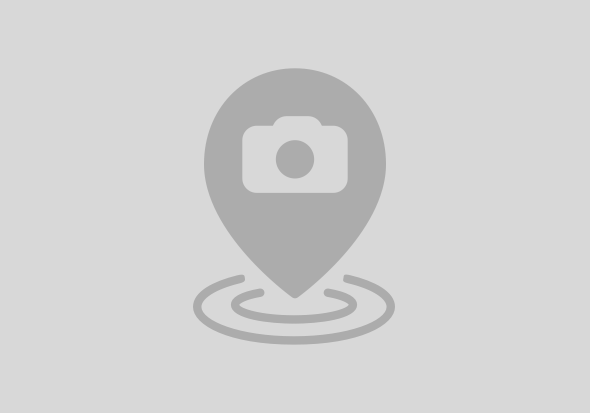
node --version
v18.12.1
npm install –global @sap/cds-dk
cds --version
mkdir captravel
cd captravel
cds init
npm install
cds watch
using { Currency, managed, sap.common.CodeList } from '@sap/cds/common';
namespace captravel;
// Travel Entity - Parent
entity Travel : managed {
key TravelUUID : UUID;
TravelID : Integer @readonly;
BeginDate : Date;
EndDate : Date;
TravelPrice : Decimal(16,2) @Measures.ISOCurrency: TravelCurrency_code;
TravelCurrency : Currency;
Description : String(255);
to_Booking : Composition of many Booking on to_Booking.to_Travel = $self;
}
// Booking Entity - Child
entity Booking: managed {
key BookingUUID : UUID;
BookingID : Integer @readonly;
BookingDate : Date;
ConnectionID : String(4);
FlightDate : Date;
FlightPrice : Decimal(16,2) @Measures.ISOCurrency: FlightCurrency_code;
FlightCurrency : Currency;
BookingStatus : Association to BookingStatus;
to_Travel : Association to Travel;
}
// Code List
entity BookingStatus : CodeList {
key Code : String enum {
New = 'N';
Booked = 'B';
Canceled = 'C';
};
};
using { captravel as my } from '../db/schema';
service TravelService {
@odata.draft.enabled //Enable Draft
entity Travel as projection on my.Travel;
entity Booking as projection on my.Booking;
}
code;name
N;New
X;Canceled
B;Booked
code;symbol;name;descr
EUR;€;Euro;European Euro
USD;$;US Dollar;United States Dollar
CAD;$;Canadian Dollar;Canadian Dollar
AUD;$;Australian Dollar;Australian Dollar
GBP;£;British Pound
ILS;₪;Shekel
INR;₹;Rupee;Indian Rupee
QAR;﷼;Riyal;Katar Riyal
SAR;﷼;Riyal;Saudi Riyal
JPY;¥;Yen;Japanese Yen
CNY;¥;Yuan;Chinese Yuan Renminbi
SGD;S$;Singapore Dollar;Singapore Dollar
ZAR;R;Rand;South African Rand
using TravelService from './service';
annotate TravelService.Travel with{
TravelID @title : 'Travel ID';
BeginDate @title : 'Begin Date';
EndDate @title : 'End Date';
TravelPrice @title : 'Price';
TravelCurrency @title : 'Currency';
Description @title : 'Description';
to_Booking @title : 'Bookings';
}
annotate TravelService.Booking with{
BookingID @title : 'Booking ID';
BookingDate @title : 'Booking Date';
ConnectionID @title : 'Connection ID';
FlightDate @title : 'Flight Date';
FlightPrice @title : 'Flight Price';
FlightCurrency @title : 'Currency';
}
annotate TravelService.Travel with @(
UI: {
HeaderInfo: {
TypeName: 'Travel',
TypeNamePlural: 'Travels',
Title : {
$Type : 'UI.DataField',
Value : TravelID
},
Description : {
$Type: 'UI.DataField',
Value: Description
}
},
SelectionFields: [TravelID],
LineItem:[
{Value: TravelID},
{Value: BeginDate},
{Value: EndDate},
{Value: TravelPrice},
{Value: Description}
],
Facets: [
{$Type: 'UI.ReferenceFacet', Label: 'Main', Target: '@UI.FieldGroup#Main'},
{$Type: 'UI.ReferenceFacet', Label: 'Flights', Target: 'to_Booking/@UI.LineItem' }
],
FieldGroup#Main: {
Data: [
{Value: TravelID},
{Value: BeginDate},
{Value: EndDate},
{Value: TravelPrice},
{Value: Description}
]
}
}
){};
annotate TravelService.Booking with @(
UI: {
LineItem: [
{Value: BookingID},
{Value: BookingDate},
{Value: ConnectionID},
{Value: FlightDate},
{Value: FlightPrice},
{Value: BookingStatus_Code, Label: 'Booking Status'}
]
}
){};
const cds = require('@sap/cds');
class TravelService extends cds.ApplicationService{
async init(){
const { Travel, Booking } = this.entities;
this.before('NEW', Travel, async req => {
const { maxID } = await SELECT.one `max(TravelID) as maxID` .from (Travel)
req.data.TravelID = maxID + 1
} )
this.before('NEW', Booking, async req => {
const { to_Travel_TravelUUID } = req.data
const { maxID } = await SELECT.one `max(BookingID) as maxID` .from (Booking.drafts) .where ({to_Travel_TravelUUID})
req.data.BookingID = maxID + 1
req.data.BookingStatus_code = 'N'
req.data.BookingDate = (new Date).toISOString().slice(0,10)
} )
await super.init()
}
}
module.exports = TravelService
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
6 | |
5 | |
5 | |
5 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 |