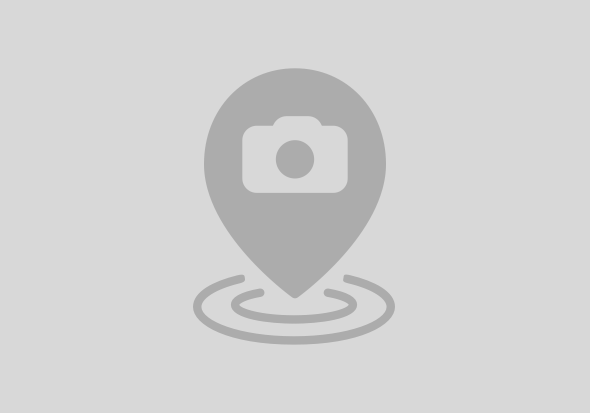
Hello Community,
In recent days, i received some tasks related to XML files via AL11. I needed examples for the conversion from XML to ABAP and from ABAP to XML, especially regarding date conversions, as I noticed there are very few resources on this topic. I decided to write this blog so that others don't lose the time I lost in finding the solution and to provide some support on this issue.
Firstly,
During my research, I found that the Global Data Types (GDT) Conversion (cl_gdt_conversion) class can be used for this purpose.
Some methods of this class can be used as follows:
To convert the time and date in ABAP format to the XML format yyyy-mm-ddThh:mm:ssTimeZone (e.g., 2024-06-05T17:16:10+02:00), the Time Outbound method can be used:
cl_gdt_conversion=>date_time_outbound(
EXPORTING
im_date = sy-datum
im_time = sy-uzeit
im_timezone = sy-zonlo
IMPORTING
ex_value = DATA(lv_xml_erstdat)
).
To convert the timestamp in the format yyyy-mm-ddThh:mm:ssTimeZone (e.g., 2024-06-05T17:26:01+02:00) to JJJJMMTThhmmss, JJJJMMTThhmmssmmmuuun, JJJJMMTT, or HHMMSS, the Time Inbound Method can be used:
cl_gdt_conversion=>date_time_inbound(
EXPORTING
im_value = lv_xml_erstdat
IMPORTING
ex_value_short = DATA(lv_abap_short)
ex_value_long = DATA(lv_abap_long)
ex_date = DATA(lv_abap_date)
ex_time = DATA(lv_abap_time)
ex_local_time = DATA(lv_abap_local)
).
Now, let's create an example. First, let's select 3 rows from the SFLIGHT table containing date fields. Let's take a field that gives the difference between the current date and the date field as a duration, and then write a program to display this as XML on the screen:
DATA: it_itab TYPE TABLE OF sflight,
secs(11) TYPE p DECIMALS 7,
negative TYPE flag,
lv_abap_duration TYPE sduration,
lv_xml_duration TYPE string,
lv_hours TYPE i,
lv_minutes TYPE i.
SELECT carrid connid fldate paymentsum FROM sflight INTO CORRESPONDING FIELDS OF TABLE it_itab UP TO 3 ROWS.
DATA(xml_schema) = |<?xml version="1.0" encoding="UTF-8"?>| &&
|<SFLIGHTS_Export>| &&
| <xs:schema id="SFLIGHTS_Export" xmlns="" xmlns:xs="http://www.w3.org/2001/XMLSchema" xmlns:msdata="urn:schemas-microsoft-com:xml-msdata">| &&
| <xs:element name="SFLIGHT_Export" msdata:IsDataSet="true" msdata:UseCurrentLocale="true">| &&
| <xs:complexType>| &&
| <xs:choice minOccurs="0" maxOccurs="unbounded">| &&
| <xs:element name="Flights">| &&
| <xs:complexType>| &&
| <xs:sequence>| &&
| <xs:element name="Carrid" type="xs:string" minOccurs="0" />| &&
| <xs:element name="Connid" type="xs:string" minOccurs="0" />| &&
| <xs:element name="Fldate" type="xs:dateTime" minOccurs="0" />| &&
| <xs:element name="Dauert" type="xs:duration" minOccurs="0" />| &&
| <xs:element name="Heute" type="xs:dateTime" minOccurs="0" />| &&
| <xs:element name="Paymentsum" type="xs:string" minOccurs="0" />| &&
| </xs:sequence>| &&
| </xs:complexType>| &&
| </xs:element>| &&
| </xs:choice>| &&
| </xs:complexType>| &&
| </xs:element>| &&
| </xs:schema>|.
DATA(xml_body) = |<flights>|.
LOOP AT it_itab INTO DATA(ls_itab).
"setting todays date as xml format
TRY.
cl_gdt_conversion=>date_time_outbound(
EXPORTING
* im_value_short = " UTC-Zeitstempel in Kurzform (JJJJMMTThhmmss)
* im_value_long = " UTC-Zeitstempel in Langform (JJJJMMTThhmmssmmmuuun)
im_date = sy-datum " Datum und Zeit, aktuelles (Applikationsserver-)Datum
im_time = sy-uzeit " Datum und Zeit, aktuelle (Applikationsserver-) Uhrzeit
* im_local_time = 'X' " Zeitstempel ist lokale Zeit, sonst UTC
im_timezone = sy-zonlo " Zeitzone
IMPORTING
ex_value = DATA(lv_xml_now) " Zeitstempel gem. Aufbau ISO 8601
).
" setting Fldate from "SFLIGHT"
cl_gdt_conversion=>date_time_outbound(
EXPORTING
* im_value_short = " UTC-Zeitstempel in Kurzform (JJJJMMTThhmmss)
* im_value_long = " UTC-Zeitstempel in Langform (JJJJMMTThhmmssmmmuuun)
im_date = ls_itab-fldate " Datum und Zeit, aktuelles (Applikationsserver-)Datum
im_time = sy-uzeit " Datum und Zeit, aktuelle (Applikationsserver-) Uhrzeit
im_local_time = 'X' " Zeitstempel ist lokale Zeit, sonst UTC
im_timezone = sy-zonlo " Zeitzone
IMPORTING
ex_value = DATA(lv_xml_datum) " Zeitstempel gem. Aufbau ISO 8601
).
*********************************************************************
" Calculating duration
cl_abap_tstmp=>td_subtract(
EXPORTING
date1 = sy-datum
time1 = sy-uzeit
date2 = ls_itab-fldate
time2 = sy-uzeit
IMPORTING
res_secs = secs ).
IF secs < 0.
negative = abap_true.
secs = - secs.
ENDIF.
lv_abap_duration-seconds = secs MOD 60.
lv_minutes = secs DIV 60.
lv_abap_duration-minutes = lv_minutes MOD 60.
lv_hours = lv_minutes DIV 60.
lv_abap_duration-hours = lv_hours MOD 24.
lv_abap_duration-days = lv_hours DIV 24.
cl_gdt_conversion=>duration_outbound(
EXPORTING
im_duration = lv_abap_duration
IMPORTING
ex_value = lv_xml_duration ).
IF negative = abap_true.
lv_xml_duration = |-{ lv_xml_duration }|.
ENDIF.
CATCH cx_gdt_conversion. " Fehler bei Global Data Types Konvertierung
ENDTRY.
xml_body = xml_body &&
|<flight>| &&
|<Carrid>| && ls_itab-carrid && |</Carrid>| &&
|<Connid>| && ls_itab-connid && |</Connid>| &&
|<Fldate>| && lv_xml_datum && |</Fldate>| &&
|<Dauert>| && lv_xml_duration && |</Dauert>| &&
|<Heute>| && lv_xml_now && |</Heute>| &&
|<Paymentsum>| && ls_itab-paymentsum && |</Paymentsum>| &&
|</flight>|.
ENDLOOP.
DATA(xml) = xml_schema && xml_body && |</flights>| && |</SFLIGHTS_Export>|.
TRY.
cl_soap_xml_helper=>xml_show(
* EXPORTING
sdoc = xml
* xdoc =
html = abap_false
* new_window =
* title = text-001
).
CATCH cx_root INTO DATA(e_txt).
WRITE: / e_txt->get_text( ).
ENDTRY.
Thank you for taking the time to read my blog. I hope it has been a useful article.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
9 | |
7 | |
7 | |
6 | |
6 | |
5 | |
5 | |
4 | |
4 | |
3 |