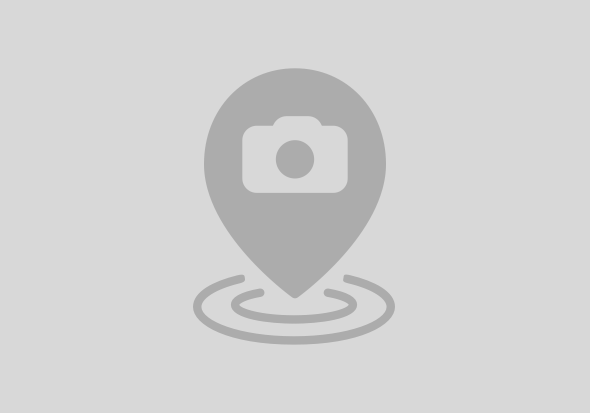
Json.IsValidJson(json_string) : Returns true for valid JSON
Json.GetArrayLength(keys, json_string): Returns the total array length for the keys passed.
Json.IsValidJson(json_string)
import ABSL;
import AP.PDI.Utilities;
var JsonString = "{\"d\": {\"results\": [{ \"__metadata\": { \"type\": \"EmployeeDetails.Employee\" }, \"UserID\": \"E12012\",\"RoleCode\": \"35\"}]}}";
var Result = Json.IsValidJson(JsonString);
KeysTable: Table consisting of keys for which value is required.
JsonString: JSON string consisting the keys passed
After executing the API, the function returns the following parameter in the resulting structure.
Key: Key passed in the importing parameter.
Value: Value obtained for the key passed.
Error: Flag indicating an error; True if there is an error, else False.
Message: Information on why the error occurred (for example, Invalid key [Key_Name], if an invalid key is passed).
Json.ParseKeyValues(keys, json_string)
import AP.PDI.Utilities;
import AP.Common.GDT;
var Result : JsonResult;
var keys : collectionof LANGUAGEINDEPENDENT_EXTENDED_Text;
var key ;
key = "d.results[1].UserID";//full path of key needs to be specified here
keys.Add(key);
key = "d.results[1].RoleCode";//full path of key needs to be specified here
keys.Add(key);
var JsonString = "{\"d\": {\"results\": [{ \"__metadata\": { \"type\": \"EmployeeDetails.Employee\" }, \"UserID\": \"E12012\",\"RoleCode\": \"35\"}]}}";
Result = Json.ParseKeyValues(keys, JsonString);
foreach(var res in Result.KeyValue)
{
var value = res.Value;
}
Key: Key passed in the importing parameter.
Length: Numeric value representing array length; -1 is sent if any error occurs or passed key is not an array.
Json.GetArrayLength(keys, json_string)
import AP.PDI.Utilities;
import AP.Common.GDT;
var Result : JsonArrayLength;
var keys : collectionof LANGUAGEINDEPENDENT_Text;
var key ;
key = "d.results";//valid key
keys.Add(key);
var JsonString = "{\"d\": {\"results\": [{ \"__metadata\": { \"type\": \"EmployeeDetails.Employee\" }, \"UserID\": \"E12012\",\"RoleCode\": \"35\"}]}}";
Result = Json.GetArrayLength (keys, JsonString);
foreach(var res in Result.Arraylength)
{
var value = res.Length;
}
JSON reuse library functions are very useful to parse JSON format.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
7 | |
6 | |
5 | |
4 | |
4 | |
4 | |
4 | |
4 | |
3 | |
3 |