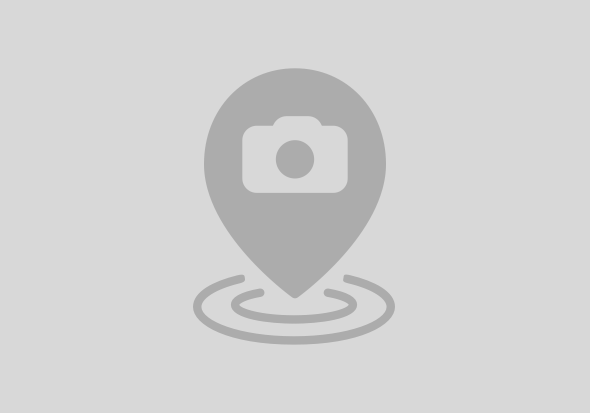
REPORT z_fetch_slack_data.
*Declarations for REST handlers
DATA: go_http_client TYPE REF TO if_http_client,
go_rest_client TYPE REF TO cl_rest_http_client.
*Declarations for Data refereces
DATA:lo_json TYPE REF TO /ui2/cl_json.
*Fetch the SLACK destination details from SM59 and create the HTTP client reference
CALL METHOD me>create_http_client
EXPORTING
i_rfc_dest = gc_rfc_dest
IMPORTING
e_return = l_wa_return.
IF l_wa_return IS NOT INITIAL.
* Send the error message
e_return = l_wa_return.
RETURN.
ENDIF.
METHOD create_http_client.
*& This method is used to fetch the destination details about the SLACK Server from SM59*
*---------------------------------------------------------------------------*
* Data Declarations*
*---------------------------------------------------------------------------*
DATA:lv_reason TYPE string,
lv_utc_timestamp TYPE timestampl.
DATA gv_auth_val TYPE string.
CONSTANTS gc_auth TYPE string VALUE 'Authorization' ##NO_TEXT.
CONSTANTS gc_content_type TYPE string VALUE 'content-type'.
CONSTANTS gc_accept TYPE string VALUE 'ACCEPT' ##NO_TEXT.
CONSTANTS gc_accept_value TYPE string VALUE 'application/json'.
CONSTANTS gc_rfc_dest TYPE rfcdest VALUE 'SLACK_REST_API_CONV' ##NO_TEXT.
CONSTANTS gc_tabname TYPE rstable-tabname VALUE 'Z_SLACK_LOG'."Table Name
CONSTANTS: gc_e TYPE dd26e-enqmode VALUE 'E'.
*Create the HTTP client instance
CALL METHOD cl_http_client=>create_by_destination
EXPORTING
destination = i_rfc_dest
IMPORTING
client = go_http_client
EXCEPTIONS
destination_not_found = 1
internal_error = 2
argument_not_found = 3
destination_no_authority = 4
plugin_not_active = 5
OTHERS = 5.
IF sy-subrc NE 0.
"Log the Exception
*Ping Destination Failed get time stamp field
lv_utc_timestamp.
lv_reason = sy-msgv1.
RETURN.
ENDIF.
CHECK go_http_client IS BOUND.
*Set the HTTP header fields
CALL METHOD go_http_client->request->set_header_field
EXPORTING
name = gc_auth
value = gv_auth_val.
CALL METHOD go_http_client->request->set_header_field
EXPORTING
name = gc_accept
value = gc_accept_value.
CALL METHOD go_http_client->request->set_header_field
EXPORTING
name = gc_content_type
value = gc_accept_value.
" set http protocol version
go_http_client->request->set_version(
if_http_request=>co_protocol_version_1_0 ) .
ENDMETHOD.
REPORT z_fetch_slack_data.
*Declations to store the native format data
TYPES: BEGIN OF ts_slack_info,
user TYPE string,
email TYPE string,
END OF ts_slack_info .
DATA ls_slack_data TYPE ts_slack_info.
DATA go_http_client TYPE REF TO if_http_client .
DATA: lr_json TYPE REF TO /ui2/cl_json.
CHECK go_http_client IS BOUND.
*Set the User ID
IF c_user_id IS NOT INITIAL.
lv_user_guid = c_user_id-zuser_guid.
cl_http_utility=>set_request_uri(
EXPORTING request = go_http_client->request uri = lv_user_guid ).
*Create REST Client object
CREATE OBJECT lo_rest_client
EXPORTING
io_http_client = go_http_client.
TRY.
lo_rest_client->if_rest_client~get( ).
DATA(lo_response) = lo_rest_client->if_rest_client~get_response_entity( ).
DATA(lv_http_status) = lo_response->get_header_field( '~status_code' ).
IF lv_http_status NE 200.
lv_error_status = lv_http_status.
*HTTP Request Failed
DATA(lv_reason) = lo_response->get_header_field( '~status_reason' ).
*STOP Processing
e_return-type = gc_err.
e_return-message = lv_reason.
RETURN.
ENDIF.
*Receive the response data in JSON.
DATA(lv_json_data) = lo_response->get_string_data( ).
"Refresh the SLACK response to clear HTTP memory of previous calls
IF go_http_client IS BOUND.
go_http_client->refresh_response( ).
"Close HTTP session (Exception HTTP_NO_MEMORY)
go_http_client->close( ).
ENDIF.
*Collect into the exception table.
CATCH cx_rest_client_exception INTO DATA(lo_rest_client_exception).
ENDTRY.
IF lv_json_data IS NOT INITIAL.
CREATE OBJECT lr_json.
TRY .
lr_json->deserialize_int( EXPORTING json = lv_json_data CHANGING data = ls_slack_data ).
ENDIF.
CATCH cx_sy_move_cast_error INTO DATA(lo_move_cast_error) .
DATA(lv_msg_desrl_err) = `HTTP GET failed: ` && lo_move_cast_error->get_longtext( ). FREE:l_wa_reason,lo_move_cast_error.
ENDTRY.
ENDIF.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
50 | |
5 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 | |
2 |